Combobox
An input that behaves similarly to a select, with the addition of a free text input to filter options.
Based on Headless UI.
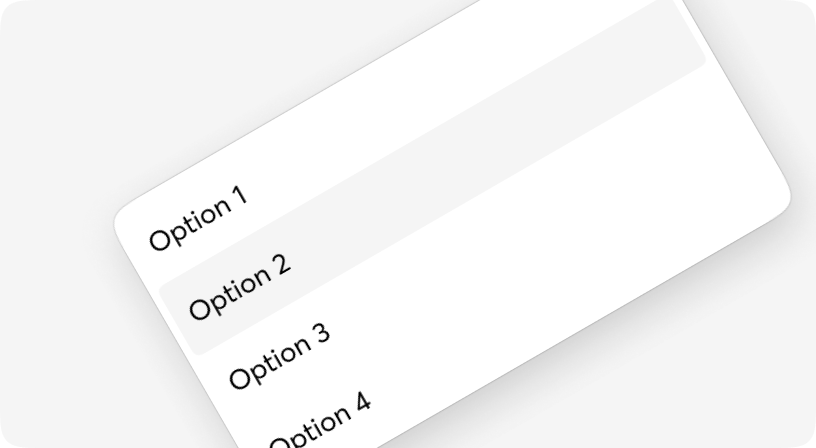
Anatomy
<Combobox> <Combobox.Trigger>...</Combobox.Trigger> <Combobox.Options> <Combobox.Option>...</Combobox.Option> </Combobox.Options> <Combobox.Hint>...</Combobox.Hint> </Combobox>
Default / Nullable
Informative message holder (default)
Informative message holder (nullable)
Anatomy
<Combobox> <Combobox.Select>...</Combobox.Select> <Combobox.Options> <Combobox.Option>...</Combobox.Option> </Combobox.Options> <Combobox.Hint>...</Combobox.Hint> </Combobox>
Select
Informative message holder
Informative message holder
Informative message holder
Different states for Select
Informative message holder
Informative message holder
Informative message holder
Informative message holder
Anatomy
<Combobox> <Combobox.InsetSelect>...</Combobox.InsetSelect> <Combobox.Options> <Combobox.Option>...</Combobox.Option> </Combobox.Options> <Combobox.Hint>...</Combobox.Hint> </Combobox>
Select with inner label
Informative message holder
Different states for Select with inner label
Informative message holder
Informative message holder
Informative message holder
Informative message holder
Anatomy
<Combobox> <Combobox.MultiSelect>...</Combobox.MultiSelect> <Combobox.Options> <Combobox.Option>...</Combobox.Option> </Combobox.Options> <Combobox.Hint>...</Combobox.Hint> </Combobox>
MultiSelect
Informative message holder
Informative message holder
Informative message holder
MultiSelect with "Select all" option
Informative message holder
Anatomy
<Combobox> <Combobox.InsetMultiSelect>...</Combobox.InsetMultiSelect> <Combobox.Options> <Combobox.Option>...</Combobox.Option> </Combobox.Options> <Combobox.Hint>...</Combobox.Hint> </Combobox>
MultiSelect with inner label
Informative message holder
Anatomy
<Combobox> <Combobox.VisualMultiSelect>...</Combobox.VisualMultiSelect> <Combobox.Options> <Combobox.Option>...</Combobox.Option> </Combobox.Options> <Combobox.Hint>...</Combobox.Hint> </Combobox>
VisualMultiSelect
Without tracking the state of the input field
When the state of the input field changes, use `forceUpdate`.
Alignment of controls for options with long names
Example with checkboxes
Example with radio buttons
Combobox
Name | Type | Required | Default | Description |
---|---|---|---|---|
value | unknown | Yes | - | The selected value. |
onChange | (value: unknown) => void | Yes | - | The function to call when a new option is selected. |
onQueryChange | (value: string) => void | Yes | - | The function to call when the filter query is changing. |
onClear | (index?: number | string) => void | No | - | The function to call when the selected options at MultiSelect Combobox type are being cleared. |
isError | boolean | No | - | Set valid/non-valid |
disabled | boolean | No | - | Set disabled/non-disabled |
size | sm | md | lg | xl | string | No | md | Size |
className | string | No | - | Tailwind classes for custom styles. |
position | top-start | top-end | bottom-start | bottom-end | right-start | right-end | left-start| left-end | top | bottom | right| left | No | bottom | Set placement for combobox |
Render Props: | ||||
open | boolean | No | - | Whether or not the Listbox is open. |
multiple | boolean | No | - | Whether multiple options can be selected or not. |
nullable | boolean | No | - | Whether the selected value can be cleared or not. |
Combobox.Trigger
Name | Type | Required | Default | Description |
---|---|---|---|---|
className | string | No | - | Tailwind classes for custom styles. |
onClose | (value: unknown) => void; | No | - | Called when the Combobox is dismissed. |
Combobox.Input | Combobox.InsetInput | Combobox.VisualSelectInput
Name | Type | Required | Default | Description |
---|---|---|---|---|
onChange | (value: unknown) => void | Yes | - | The function to call when a new option is selected. |
onQueryChange | (value: string) => void | Yes | - | The function to call when the query filter is changing. |
displayValue | (value: unknown) => string | No | - | The value of the selected option to display. |
label | string | undefined | No | - | Inset label title (for InsetInput) |
placeholder | string | undefined | No | - | Placeholder |
type | text | string | number | date | time | date-time-local | undefined | No | text | Type of the input field |
className | string | No | - | Tailwind classes for custom styles. |
Combobox.Button
Name | Type | Required | Default | Description |
---|---|---|---|---|
className | string | No | - | Tailwind classes for custom styles. |
Render Props: | ||||
open | boolean | No | - | Whether or not the Listbox is open. |
Combobox.Options
Name | Type | Required | Default | Description |
---|---|---|---|---|
menuWidth | string | No | - | Tailwind class for custom options container width. |
className | string | No | - | Tailwind classes for custom styles. |
Combobox.Option
Name | Type | Required | Default | Description |
---|---|---|---|---|
value | unknown | No | - | The option value. |
Render Props: | ||||
active | boolean | No | - | Whether or not the option is the active/focused option. |
selected | boolean | No | - | Whether or not the option is the selected option. |
Combobox.Transition
Name | Type | Required | Default | Description |
---|---|---|---|---|
onQueryChange | (value: string) => void | Yes | - | The same function that is set in the Combobox component. |
Combobox.Select | Combobox.InsetSelect
Name | Type | Required | Default | Description |
---|---|---|---|---|
onChange | (value: unknown) => void | Yes | - | The function to call when a new option is selected. |
onQueryChange | (value: string) => void | Yes | - | The function to call when the query filter is changing. |
displayValue | (value: unknown) => string | No | - | The value of the selected option to display. |
label | JSX.Element | string | No | - | Label title | Inset label title |
placeholder | JSX.Element | string | No | - | Placeholder |
className | string | No | - | Tailwind classes for custom styles. |
Render Props: | ||||
open | boolean | No | - | Whether or not the Listbox is open. |
onClose | (value: unknown) => void; | No | - | Called when the Combobox is dismissed. |
Combobox.MultiSelect | Combobox.InsetMultiSelect | Combobox.VisualMultiSelect
Name | Type | Required | Default | Description |
---|---|---|---|---|
onChange | (value: unknown) => void | Yes | - | The function to call when a new option is selected. |
onQueryChange | (value: string) => void | Yes | - | The function to call when the query filter is changing. |
displayValue | (value: unknown) => string | No | - | The value of the selected option to display. |
label | JSX.Element | string | No | - | Label title | Inset label title |
placeholder | JSX.Element | string | No | - | Placeholder |
className | string | No | - | Tailwind classes for custom styles. |
counter | Number | No | 0 | Number of selected options |
Render Props: | ||||
open | boolean | No | - | Whether or not the Listbox is open. |
onClose | (value: unknown) => void; | No | - | Called when the Combobox is dismissed. |
Combobox.VisualMultiSelect
Name | Type | Required | Default | Description |
---|---|---|---|---|
forceUpdate | boolean | No | - | If you need to align the list of options properly on each select/deselect set the "boolean" property. |
Combobox.Counter
Name | Type | Required | Default | Description |
---|---|---|---|---|
counter | number | Yes | - | Number of selected options |
className | string | No | - | Tailwind classes for custom styles. |
Render Props: | ||||
open | boolean | No | - | Whether or not the Listbox is open. |
Combobox.SelectedItem
Name | Type | Required | Default | Description |
---|---|---|---|---|
index | number | string | Yes | - | Index of the selected item |
label | number | string | Yes | - | Displayed label of the selected item |
className | string | No | - | Tailwind classes for custom styles. |
Render Props: | ||||
open | boolean | No | - | Whether or not the Listbox is open. |
Combobox.Hint
Name | Type | Required | Default | Description |
---|---|---|---|---|
className | string | No | - | Tailwind classes for custom styles. |